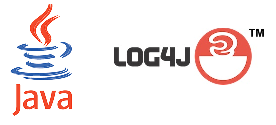
Logs are a essential part of any serious application running in the production environment, because they can trace all the users and system actions, allowing if needed, find who did what and when, or why the application crashed as well. That's the Log4j2 library mission! Here you can see how use this library with Eclipse IDE and Maven.
The Log4j2 has a lot of options, but I will show just the basic usage: how to print logs in the console and files. First let's create a new project, go to File->New->Java Project, place a name and click Finish:
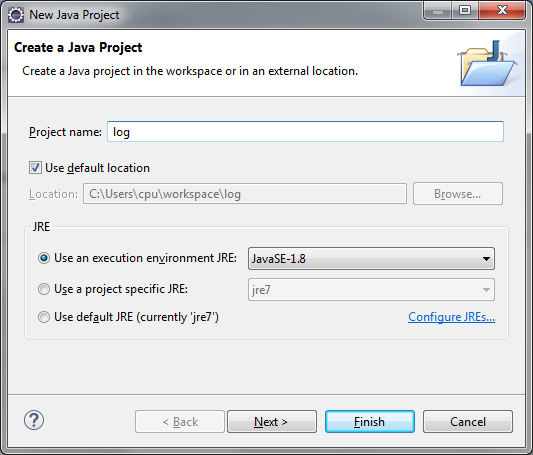
Create a new package and give as name "l3oc.com":

Create a new class and give as name "Main":
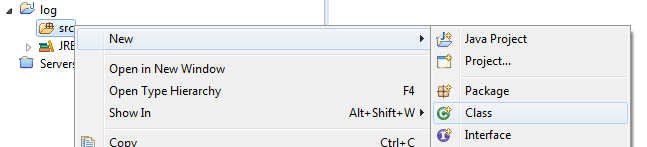
And you should have this:
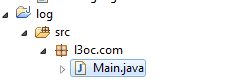
Now the Maven part, if you don't know what is Maven click here. Convert the project to a Maven project doing this:
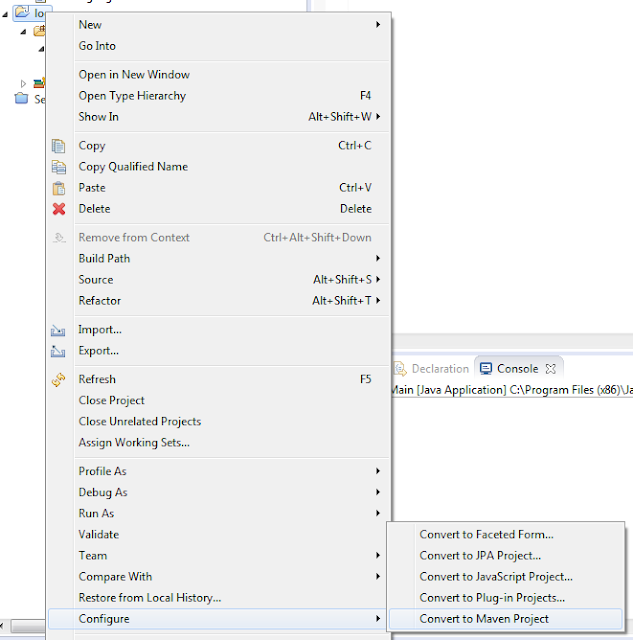
This will create the "pom.xml" file in the project, that's the Maven configuration file, now insert this Log4j2 dependency code in it (can be between version and build tags):
<dependencies> <!-- log4j 2.1 --> <dependency> <groupId>org.apache.logging.log4j</groupId> <artifactId>log4j-api</artifactId> <version>2.1</version> </dependency> <dependency> <groupId>org.apache.logging.log4j</groupId> <artifactId>log4j-core</artifactId> <version>2.1</version> </dependency> </dependencies>
And place this code inside the "Main" class:
static Logger log = LogManager.getLogger(Main.class.getName()); public static void main(String[] args) { log.trace("trace"); log.debug("debug"); log.info("info"); log.warn("warn"); log.error("error"); log.fatal("fatal"); }
The code creates the "main" method, where the code runs, with some log commands and the log object from the Logger class with the current class name. As you can see, there are levels of logs, which goes from lower to higher level, in the following order (like in the code): trace < debug < info < warn < error < fatal .
Now the Log4j2 configuration file, create a file named "log4j2.xml" in the "src" directory with this content:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE xml> <Configuration status="warn" name="MyApp" packages=""> <Appenders> <File name="File" fileName="logfile.log"> <PatternLayout pattern="%d{dd/MM/yyyy - HH:mm:ss} | %-5level | %logger{36} -> %M() -> %msg%n" /> </File> <Console name="Console" target="SYSTEM_OUT"> <PatternLayout pattern="%d{dd/MM/yyyy - HH:mm:ss} | %-5level | %logger{36} -> %M() -> %msg%n" /> </Console> </Appenders> <Loggers> <logger name="l3oc.com" level="ALL" additivity="false"> <AppenderRef ref="File" level="INFO" /> <AppenderRef ref="Console" level="ALL"/> </logger> <Root level="ERROR"> </Root> </Loggers> </Configuration>
What the hell this mean? the "logger" tag will just look the l3oc.com.* files and log all levels (ALL), using 2 ways (appenders), "File" and "Console". The "File" will log just the INFO and higher levels (info,warn,error and fatal) in a file named "logfile.log", in the project directory. The "Console" will log ALL log levels. The "pattern" syntax and its details can be found here.
The video below show the result:
About the versions
- Eclipse Java EE IDE for Web Developers Luna Service Release 1 (4.4.1)
- Log4j2 2.1
0 comentários :
Post a Comment