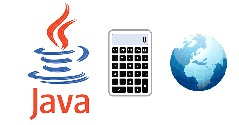
If you don't know some term or technology used in the post, click here. Start creating a new "Dynamic Web Project":

Choose the project name, Tomcat server and click "Next":
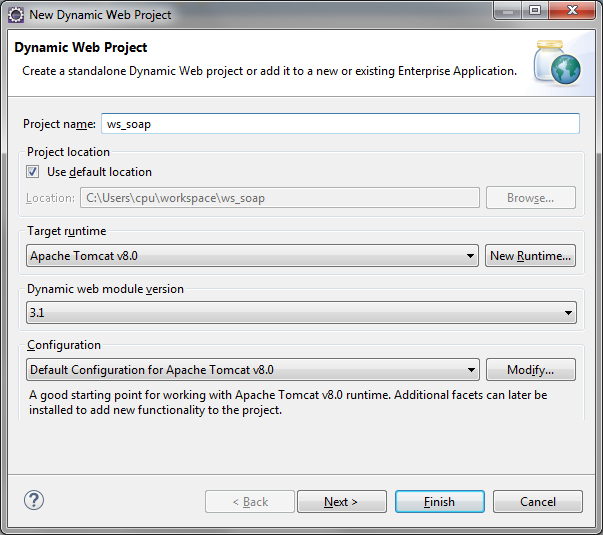
Set the checkbox to create the "web.xml" file and then click "Finish":
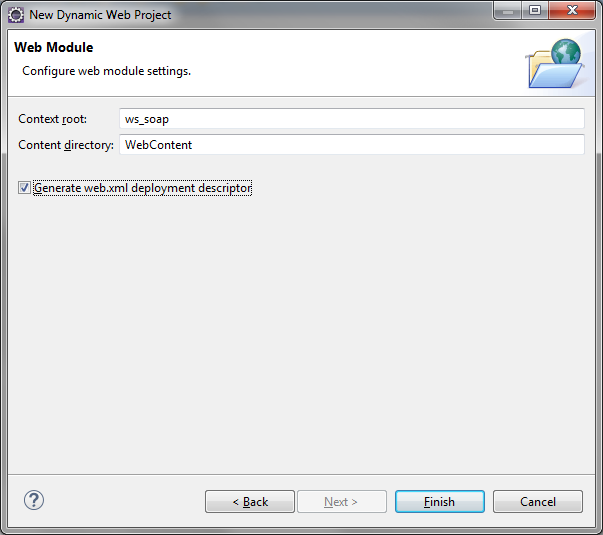
Insert the code below in the "web.xml" file inside the "web-app" tag:
<listener> <listener-class> com.sun.xml.ws.transport.http.servlet.WSServletContextListener </listener-class> </listener> <servlet> <servlet-name>calculator</servlet-name> <servlet-class> com.sun.xml.ws.transport.http.servlet.WSServlet </servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>calculator</servlet-name> <url-pattern>/calc</url-pattern> </servlet-mapping> <session-config> <session-timeout>30</session-timeout> </session-config>
In brief, this code is telling to the server (Tomcat) that a servlet named "calculator" will run on "/calc" URI. Now let's do the same for the JAX-WS RI configuration file, create a file named "sun-jaxws.xml" in the same directory of "web.xml" with this content:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE xml> <endpoints xmlns="http://java.sun.com/xml/ns/jax-ws/ri/runtime" version="2.0"> <endpoint name="CalculatorImpl" implementation="com.l3oc.CalculatorImpl" url-pattern="/calc" /> </endpoints>
This code is telling to the JAX-WS RI that an endpoint named "CalculatorImpl", which implementation/code is on "com.l3oc" package, class "CalculatorImpl", will handle the "/calc" web service. Now create the package "com.l3oc" in the "src" folder and a class named "Calculator" with this content:
package com.l3oc; import javax.jws.WebMethod; import javax.jws.WebService; import javax.jws.soap.SOAPBinding; import javax.jws.soap.SOAPBinding.Style; @WebService @SOAPBinding(style = Style.RPC) public interface Calculator { @WebMethod float sum(float num1, float num2); @WebMethod float subtract(float num1, float num2); @WebMethod float multiply(float num1, float num2); @WebMethod float divide(float num1, float num2); };
This class is an interface that describes the Calculator web service methods, pay attention to the annotations used, now let's implement this interface creating a class named "CalculatorImpl" with this content:
package com.l3oc; import javax.jws.WebService; @WebService(endpointInterface = "com.l3oc.Calculator") public class CalculatorImpl implements Calculator { public float sum(float num1, float num2) { return num1 + num2; } public float subtract(float num1, float num2) { return num1 - num2; } public float multiply(float num1, float num2) { return num1 * num2; } public float divide(float num1, float num2) { return num1 / num2; } }
This class has all the four web service methods, where each one has two numbers as input, all float values and returns the requested math operation result. Now The web service is ready to test! build the project and run:
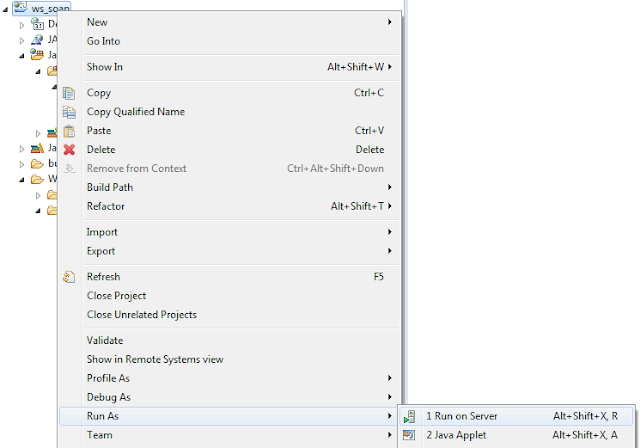
The Eclipse built-in browser should open with the wrong URL, just correct it to "http://localhost:8080/ws_soap/calc" or with your server address, and you should see something like this:
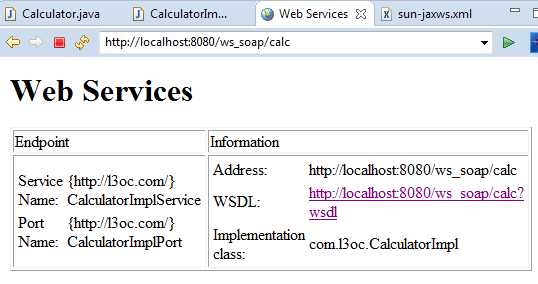
Now click in the WSDL link to see the web service details. That's all! we can't test the methods without a client requesting them, so let's create the client !
Download
About the versions
- Eclipse Java EE IDE for Web Developers Luna Service Release 1 (4.4.1)
- Tomcat Server 8.0
- JAX-WS RI 2.2.8
0 comentários :
Post a Comment