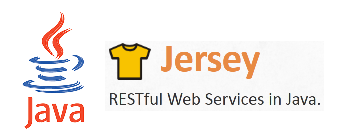
If you don't know some term or technology used in the post, click here.
Like on the Java SOAP Web Service, let's do the same calculator but just with the sum method, and now as a REST instead of SOAP web service. Start creating a new "Dynamic Web Project" and in the "web.xml" file insert this code:
<servlet> <servlet-name>calculator</servlet-name> <servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class> <init-param> <param-name>jersey.config.server.provider.packages</param-name> <param-value>com.l3oc</param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>calculator</servlet-name> <url-pattern>/rest/*</url-pattern> </servlet-mapping>
In brief, this code tells to the server (Tomcat) that a servlet named 'calculator' will run on the "/rest/*" URL, which will be handled by the Jersey library. Different from the JAX-WS RI library, that is already in the Tomcat 8 default libraries, the JAX-RS RI (Jersey) isn't, so convert the project to a Maven project and insert this dependency code in the "pom.xml" file:
<dependencies> <dependency> <groupId>org.glassfish.jersey.containers</groupId> <artifactId>jersey-container-servlet</artifactId> <version>2.15</version> </dependency> </dependencies>
Now create a package named "com.l3oc" and a class named "Calculator" with this code:
package com.l3oc; import javax.ws.rs.GET; import javax.ws.rs.Path; import javax.ws.rs.Produces; import javax.ws.rs.QueryParam; import javax.ws.rs.core.MediaType; @Path("/calc") public class Calculator { @GET @Path("/sum") @Produces(MediaType.TEXT_PLAIN) public String Sum(@QueryParam("x") int x,@QueryParam("y") int y) { return "Sum: " + x + "+" + y + "=" + (x+y); } }
This code creates "/calc" as the base web service path and "/sum" as the "Sum" method service path, that receives two parameters: "x" and "y", which will be added and return a string with the result. The result produces a text-plain response, however, you can implement the same method with different MediaType, this allows different kind of answers, like XML, HTML or JSON for example.
That's it! it's ready to test, just invoke with the following URL from your browser:
<server:port><project><servlet URI><web service URI><method URI><method parameters>
http://127.0.0.1:8080/rest/rest/calc/sum?x=2&y=3
The video below show the result:
Now try a better approach using the math operators directly on the URI! take a look here.
Download
About the versions
- Eclipse Java EE IDE for Web Developers Luna Service Release 1 (4.4.1)
- Tomcat Server 8.0
- JAX-RS Jersey version 2.15
0 comentários :
Post a Comment